Refactoring my personal website, Part 3: Share via social icons
2022-09-14
- Dev-Blog
- Personal-website
- Frontend
- Web-Development
Ultimately the goal of any content creator is to have their content reach as many people as possible. To help further this motive, one can enable their readers to share articles on social media.
This article will guide you through setting up buttons with which users can share content on social media, shown in an exemplary way for Facebook, LinkedIn and Twitter. Thereby, I will make use of Liquid, which is integrated in Jekyll to make the code as re-usable and modular as possible.
Including icons from Font Awesome
The quickest and simplest way to include icons for social media or other brands in a website, is to use Font Awesome.
Create an account and set up a kit, the Font Awesome provided cdn. Then follow the instructions
and include the script
snippet in the head
of every page that
you want to use icons on.
Once this is done you can search for the icons that you want to use here. If you then copy that icon's code snippet, that is generated for you when you click on a specific icon, into your HTML you should see the icon.
<i class="fa-brands fa-linkedin"></i>
Icons not loading in development environment
When I first set up Font Awesome in this project, the included icons did not show, even though I had set everything up as described above.
When building and serving a Jekyll project locally using bundle exec
jekyll serve
the following output is generated:
Server address: http://127.0.0.1:4000
Server running... press ctrl-c to stop.
The project is served under 127.0.0.1:4000
, so far so good.
When I did some digging in the Font Awesome kit settings, while trying to fix this issue,
I tried to manually add localhost
to the enabled domains and got
the following error message.
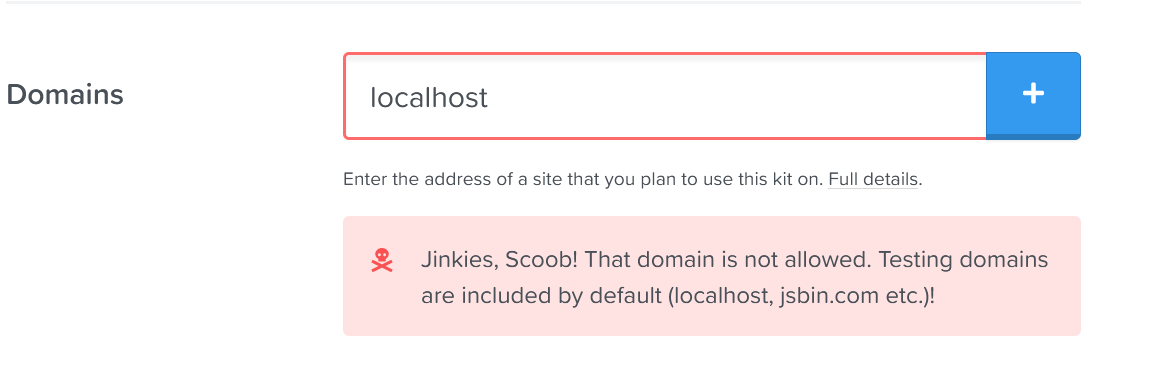
localhost
in Font Awesome Kit
localhost
is supposedly included by default, so the icons should be served
under 127.0.0.1:4000
, which is just an alias for localhost:4000
.
However, the exact wording is of importance here. Font Awesome kits only serve icons
for localhost
and not for 127.0.0.1
. Simply opening the
project served by Jekyll via localhost
fixed the problem.
HTML setup and styling
To include the social icons into the website I again made use of flexbox. The following HTML and CSS snippets yield the social icon bar that you can see at the bottom of this page.
<div id="socials">
<p>Share article via </p>
<!-- Note that these icons (<i> tags)
will turn into svgs at runtime -->
<a>
<i class="fa-brands fa-linkedin"></i>
</a>
<a>
<i class="fa-brands fa-square-twitter"></i>
</a>
<a>
<i class="fa-brands fa-square-facebook"></i>
</a>
</div>
#socials {
display: flex;
flex-flow: row;
align-items: center;
}
#socials a {
color: black;
}
#socials a:first-of-type {
color: black;
margin-left: .3rem;
}
#socials svg {
font-size: 2rem;
margin: 0 .2rem 0 .2rem;
}
One important thing to note here is that Font Awesome allows you to choose how the icons in your kit are served, by default they are served as Web Font.

If you do not change this option, your icons will be served as Web Font and you can style
your icons directly the way you included them via the provided classes and the <i>
-tag.
However, if you change this setting to SVG, the icons will be served as SVGs, meaning you need to style
them via the <svg>
-tag, even though in your code the icons are still included via
the <i>
-tag.
By inspecting the website's code you can get a clearer picture of what is happening.

<i>
-tag is replaced by an
SVG at runtime
This is also the reason why I'm using #socials svg
instead of #socials i
in the above CSS snippet.
Increasing modularity via Liquid
The only thing that is missing, is for the buttons to actually share the article on the selected social network. So the first thing that needs to be figured out is how the social media networks, for which we want to provide the ability to share content, structure their share URLs.
For this, I found a very helpful github repository which provides up-to-date documentation on how share URLs for any large social media platform work.
We can see that out of the three social media platforms, for which I'd like to provide the ability to share,
Twitter and LinkedIn use the same, single parameter url
. Twitter allows for some more customization.
Facebook:
https://www.facebook.com/sharer.php?u={url}
LinkedIn:
https://www.linkedin.com/sharing/share-offsite/?url={url}
Twitter:
https://twitter.com/intent/tweet?url={url}
&text={title}&via={user_id}&hashtags={hash_tags}
To make the share buttons as modular and re-usable as possible I have utilized the variables that Jekyll exposes to any file that uses front matter, which is any file on this website and thus blog. This has to do with maintenance and SEO reasons, but I will elaborate on those subjects in separate blog posts.
A documentation of variables that Jekyll exposes via front matter can be found here.
The first parameter to extract from these Jekyll variables is url
, as it is used by every social network.
This parameter can be extracted by accessing the variables site.url
and page.url
. The latter
resolves to the URL of a page or post without the domain e.g. /2022/12/14/my-post.html
and the former resolves
to the domain as configured in _config.yml.
If you do not yet have a _config.yml
file, create one now. Then add an entry for the URL/domain of your website.
url: https://tobias.lindenbauer.me
By accessing these variables, the share URLs can be specified as follows:
<div id="socials">
<p>Share article via </p>
<!-- Note that these icons (<i> tags)
will turn into svgs at runtime -->
<a href="https://www.linkedin.com/sharing/share-offsite/
?url={{site.url}}{{page.url}}"
target="_blank" title="Share on LinkedIn">
<i class="fa-brands fa-linkedin"></i>
</a>
<a href="http://twitter.com/share?url=
{{site.url}}{{page.url}}"
target="_blank" title="Share on Twitter">
<i class="fa-brands fa-square-twitter"></i>
</a>
<a href="https://facebook.com/sharer.php?u=
{{site.url}}{{page.url}}"
target="_blank" title="Share on Facebook">
<i class="fa-brands fa-square-facebook"></i>
</a>
</div>
Additional parameters for Twitter
As you might have noticed before, the share URL for Twitter allows for some additional
parameters: user_id
, hash_tags
and title
.
In my share buttons I made use of the latter to jazz up the shared tweet a bit more.
To realize this I made use of Front Matter, that comes with Jekyll and allows specification and usage of variables on a per page and global level. I will make use of both of these, but first, let's focus on the per page level.
To set page specific variables, at the top of a page, a section enclosed by three hyphens, is specified. Within this section page level variables can be configured.
I would like to include the title of a given
article within the tweet. Since the title differs based on the article, it has to be set per page.
Variables specified in this way can be accessed via
{{page.variable}}
, on the page on
which they were specified.
---
title: "Refactoring my personal website, Part 3:
Share via social icons"
---
I would also like to include a call to action in the generated tweets, which is some boilerplate text that intends to motivate users to engage with the tweet. This text can be the same accross all generated tweets, thus it should be specified at a global level.
To accomplish this a new variable is introduced in _config.yml
. Variables specified in
this manner can be accessed via
{{site.variable}}
in any page's HTML
source code.
twitter_cta: "Check out this article by @Liqsxd: "
Now all that is left to do, is to include these variables in the URL that is used to share an article via twitter.
<a href="http://twitter.com/share?url=
{{site.url}}{{page.url}}
&text={{site.twitter_cta}}'{{page.urlsafe_title}}'"
rel="nofollow" target="_blank" title="Share on Twitter">
<i class="fa-brands fa-square-twitter"></i>
</a>
URL safe encoding
If you have a keen eye you might have noticed that the text that is to be embedded within the share URL contains spaces. Sadly, Jekyll does not automatically encode these spaces in a URL safe manner, thus this has to be taken care of manually.
For this, simply replace all the spaces in both twitter_cta
and title
with the URL safe encoding of a space %20
.
twitter_cta: "Check%20out%20this%20article%20by%20@Liqsxd:%20"
title: "Refactoring%20my%20personal%20website,
%20Part%203:%20Share%20via%20social%20icons"
Conclusion
In this article I showed you how to setup social sharing buttons from the ground up and taught you some nice tricks, that can be utilize to keep them as re-usable and maintainable as possible, while adding in customization wherever possible.
If you enjoyed this article or found it helpful, you might want to check out my other articles and look forward to the next article in this blog series: "Refactoring my personal website, Part 4: Webhosting with Gitlab pages".
Share article via